September 23, 2024 by Slint Team
Slint 1.8 Released with New Property Changed Callbacks, Timer, and Swipe Gesture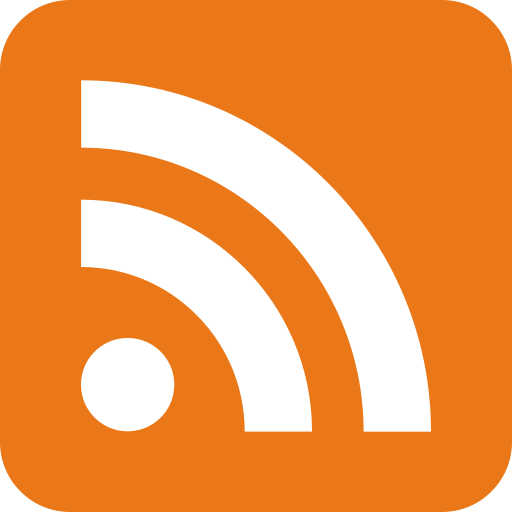
Slint is a native GUI toolkit written in Rust, with APIs in Rust, C++, Python, and JavaScript, designed for fast and responsive applications on all platforms. Today, we've released 1.8! This version brings several much requested features. Property change callbacks. Timer and SwipeGestureHandler elements. Improvements to the live-preview and vscode extension. C++ templates for STM32 embedded boards. Math gains postfix support and atan2.
Property Change Callbacks
Property bindings are part of how you can achieve a lot with so little code in Slint. Then many elements have
callbacks
to help manage side effects. Take TextEdit and the edited
call back to let you react to the
changing text the user is
being entered. However you quickly find places where your UI is updating and changing, but unlike TextEdit
there is no
callback to use. Enter Property Change Callbacks
. They can help you make your interface even more
dynamic without
having to write extra business side logic.
Let's imagine TextEdit didn't have an edited callback. You can create the same behavior as follows:
my-edit := TextEdit {
changed text => { t.text = self.text; }
}
t := Text {}
This callback is triggered when the text
property of the TextEdit
changes,
updating the text in the second Text
element accordingly.
They also can be used to help you debug your bindings.
my-edit := TextEdit {
changed text => { debug(self.text); }
}
And now see a log of every time the text changes. With great power, comes great responsibility. In most cases try to rely on declarative property bindings, which are more efficient. So be careful not to introduce unintended performance issues or loops, especially if changes cascade into other properties. For more detailed information about the caveats, check out Olivier's recent blog post.
Timer Element
Chances are at some point your user interface needs to trigger a callback at a regular interval. Now you can
easily do
this with the Timer
element.
Timer {
interval: 1s;
triggered() => { debug("Tick"); }
}
Be sure to bind to the Timer's running
property to stop the timer when you don't need it
anymore. Your battery and CPU
will thank you.
SwipeGestureHandler Element
For those needing advanced interactions we are pleased to be able
to take our gesture
system to the next level with the new SwipeGestureHandler
element. As the name suggests it
allows your UI to
recognize and respond to interactive swipe gestures.
sgh := SwipeGestureHandler {
handle-swipe-left: true;
handle-swipe-right: true;
swiped => { /* the swipe is finished */ }
}
Rectangle {
x: sgh.swiping ? sgh.current-position.x - sgh.pressed-position.x : 0px;
}
Enhanced Live Preview
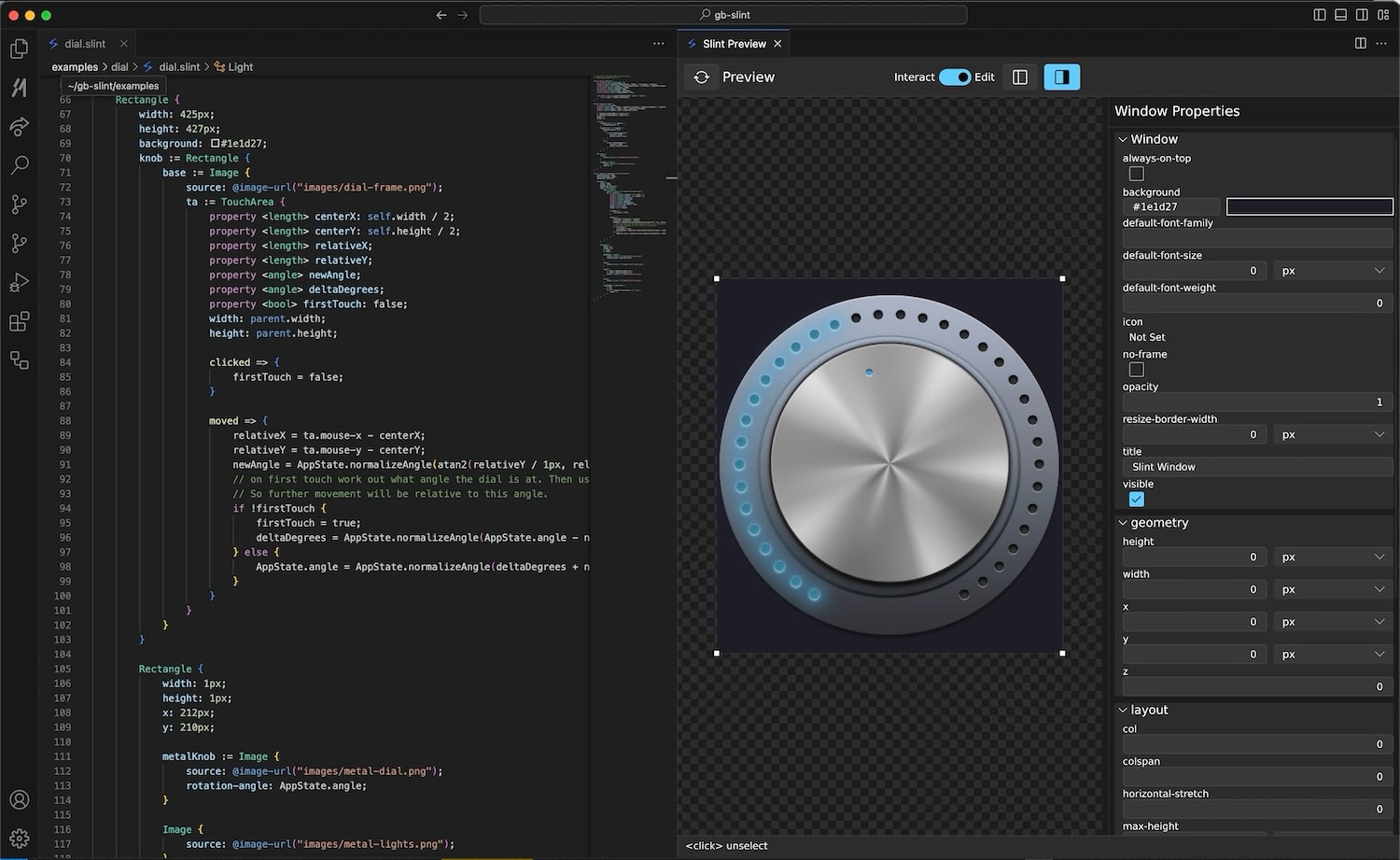
We've again improved the UX of the live-preview and enhanced the property editors.
VSCode New Project Template
If you have ever wanted to quickly create a new Slint project to try out an idea it's now easier than ever. Hit CTRL+shift+P (CMD+shift+P on Mac) and "Slint: Create New Project from Template" is a new option. It will guide you through selecting a template to fit the language of your choice.
Easier MCU Development
Are you targeting STMicroelectronics' STM32 platform with C++? In this release we've included two brand new templates that make it very easy to get started on the STM32H747I-DISCO and the STM32H735G-DK boards: 1. Download the template archive from the table in our documentation. 2. Open the extracted folder in VS Code. 3. Press F5 to cross-compile, flash, and debug in one go. We're working on adding templates for additional boards in the future. For Espressif's ESP-IDF platform with C++, we've updated our ESP-IDF component to use pre-compiled Slint binaries. This speeds up compilation time and eliminates the need to install Rust.
Math Gains Postfix Support
A subtle but profound change to the language. Traditional syntax:
Math.max(20, Math.abs(value.x))
New postfix syntax:
value.x.abs().max(20)
The new syntax improves readability by making the transformation steps more explicit. It works well for many
operations
but has limitations:
Effective for simple transformations (e.g., abs, max)
Less intuitive for operations like clamp or atan2.
pos.y.atan2(pos.x) // Less clear than atan2(pos.y, pos.x)
So for now you cannot use postfix for all functions in the Math namespace. We may revisit these cases later,
so give them a try and let us know your thoughts.
Math Gains atan2()
While creating the new dial example our new designer, Nigel, complained it would have been easier with Math.atan2. So we suggested he add it himself. It's his first time writing any Rust, so he insisted we mention that in the release notes. Time will tell if this leads to fewer complaints or more Rust commits!
Finally...
To start using Slint 1.8, you can upgrade by following the instructions attached to the GitHub release.
With this release, we hope to make Slint even more powerful and user-friendly for developers building modern, performant UIs. Be sure to check out the full ChangeLog for more details. As always, we welcome your feedback and contributions. In particular, thanks to the following contributors! @crai0 @Enyium @DataTriny @madsmtm @otiv-wannes-vanleemput @wetheredg @sigmaSd @danutsu @asuper0 @dragonrider7225 @tiaoxizhan @algovoid @0x6e @Justyna-JustCode @MiKom @botent @microhobby
Happy coding!
Slint is a Rust-based toolkit for creating reactive and fluent user interfaces across a range of targets, from embedded devices with limited resources to powerful mobile devices and desktop machines. Supporting Android, Windows, Mac, Linux, and bare-metal systems, Slint features an easy-to-learn domain-specific language (DSL) that compiles into native code, optimizing for the target device's capabilities. It facilitates collaboration between designers and developers on shared projects and supports business logic development in Rust, C++, JavaScript, or Python.